Python Raise Error and Ask Again
Watch Now This tutorial has a related video course created by the Real Python team. Watch it together with the written tutorial to deepen your understanding: Raising and Treatment Python Exceptions
A Python plan terminates as soon as it encounters an error. In Python, an error tin can be a syntax error or an exception. In this commodity, you will come across what an exception is and how it differs from a syntax error. Afterward that, yous will acquire about raising exceptions and making assertions. Then, you'll finish with a sit-in of the endeavor and except block.
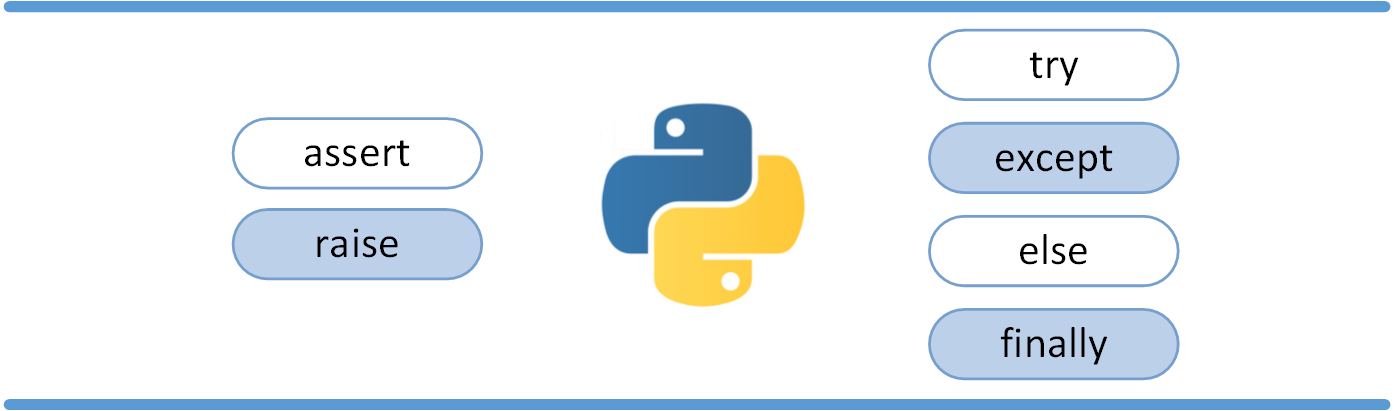
Exceptions versus Syntax Errors
Syntax errors occur when the parser detects an incorrect statement. Observe the following example:
>>> print( 0 / 0 )) File "<stdin>", line ane print ( 0 / 0 )) ^ SyntaxError: invalid syntax
The arrow indicates where the parser ran into the syntax error. In this example, there was one bracket too many. Remove it and run your code again:
>>> print( 0 / 0) Traceback (about recent call final): File "<stdin>", line 1, in <module> ZeroDivisionError: integer partitioning or modulo past goose egg
This fourth dimension, y'all ran into an exception error. This type of error occurs whenever syntactically correct Python lawmaking results in an error. The concluding line of the message indicated what type of exception error you ran into.
Instead of showing the bulletin exception error
, Python details what type of exception error was encountered. In this case, it was a ZeroDivisionError
. Python comes with various built-in exceptions equally well as the possibility to create self-defined exceptions.
Raising an Exception
Nosotros tin utilize raise
to throw an exception if a status occurs. The statement tin be complemented with a custom exception.
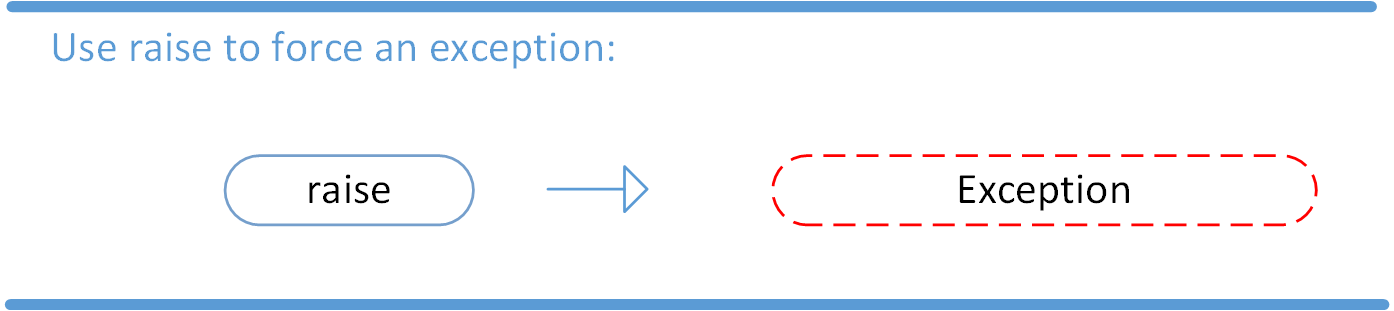
If you want to throw an mistake when a certain condition occurs using raise
, you lot could get about it like this:
ten = x if x > 5 : raise Exception ( 'ten should not exceed five. The value of x was: {} ' . format ( x ))
When you run this code, the output will be the following:
Traceback (most contempo call final): File "<input>", line iv, in <module> Exception: x should non exceed 5. The value of ten was: ten
The programme comes to a halt and displays our exception to screen, offer clues near what went wrong.
The AssertionError
Exception
Instead of waiting for a plan to crash midway, you tin can besides first past making an assertion in Python. Nosotros affirm
that a certain condition is met. If this status turns out to be True
, then that is splendid! The program tin continue. If the condition turns out to be False
, you tin have the program throw an AssertionError
exception.
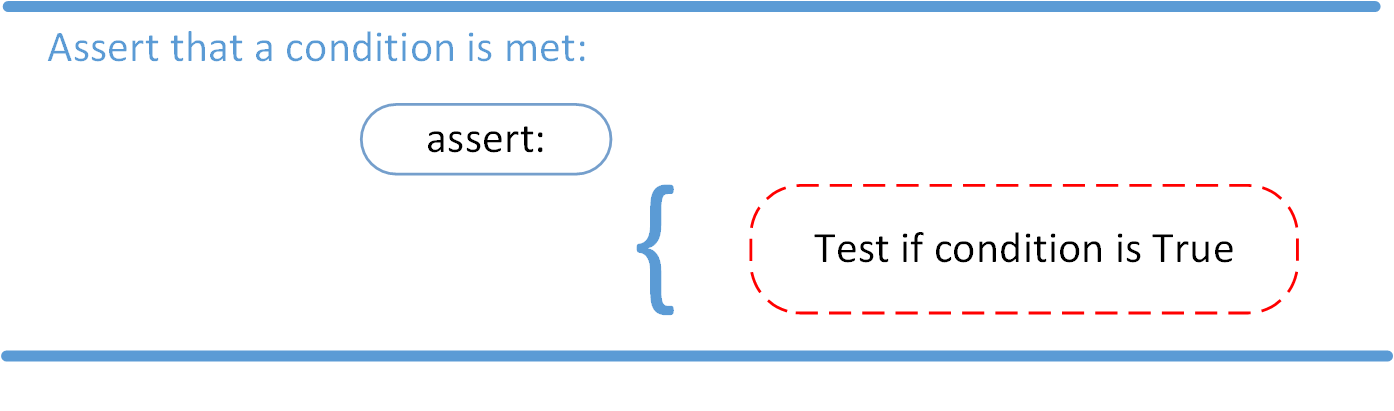
Have a look at the following example, where it is asserted that the code will be executed on a Linux system:
import sys affirm ( 'linux' in sys . platform ), "This code runs on Linux only."
If you run this lawmaking on a Linux machine, the assertion passes. If you were to run this code on a Windows machine, the outcome of the exclamation would be False
and the result would be the following:
Traceback (most recent call last): File "<input>", line 2, in <module> AssertionError: This code runs on Linux only.
In this example, throwing an AssertionError
exception is the last thing that the program will do. The program will come to halt and volition not continue. What if that is not what y'all want?
The try
and except
Cake: Handling Exceptions
The try
and except
block in Python is used to take hold of and handle exceptions. Python executes code following the endeavour
statement as a "normal" role of the programme. The code that follows the except
argument is the programme's response to any exceptions in the preceding effort
clause.
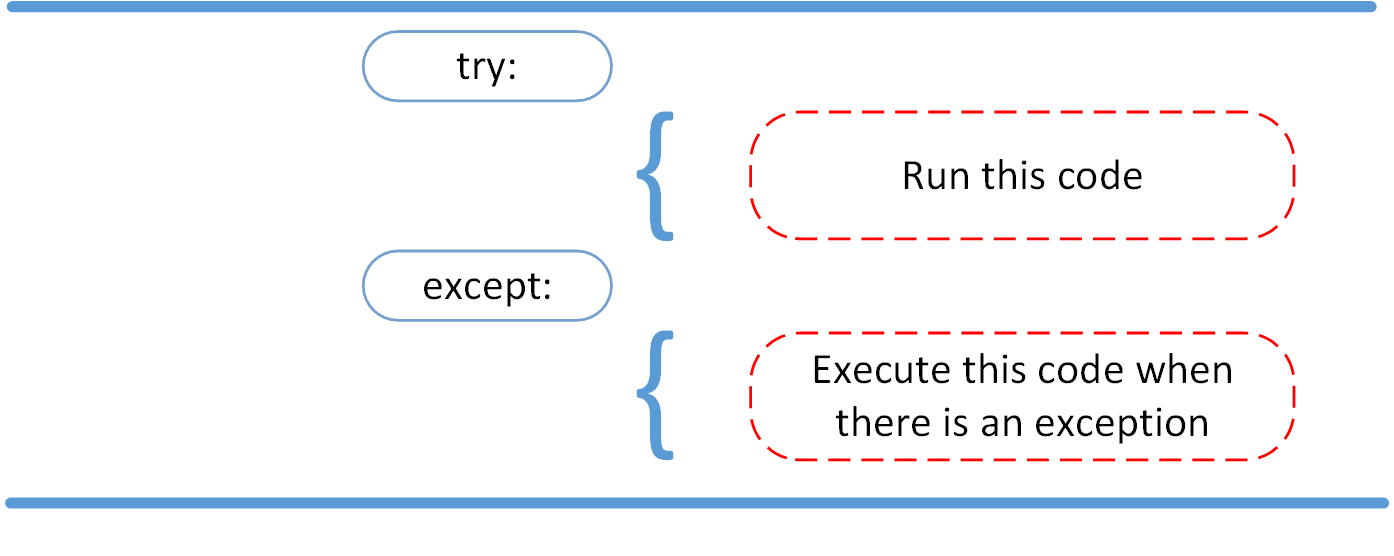
Equally you saw earlier, when syntactically correct code runs into an error, Python will throw an exception fault. This exception mistake will crash the program if it is unhandled. The except
clause determines how your plan responds to exceptions.
The following office tin assistance you understand the try
and except
block:
def linux_interaction (): assert ( 'linux' in sys . platform ), "Part can only run on Linux systems." impress ( 'Doing something.' )
The linux_interaction()
can only run on a Linux organisation. The assert
in this office will throw an AssertionError
exception if you call it on an operating system other then Linux.
You can give the function a try
using the following code:
effort : linux_interaction () except : pass
The mode you handled the error here is by handing out a pass
. If you were to run this lawmaking on a Windows machine, you would get the following output:
You got naught. The expert affair here is that the program did not crash. But it would be prissy to run into if some blazon of exception occurred whenever you ran your code. To this stop, you can change the pass
into something that would generate an informative message, like so:
try : linux_interaction () except : print ( 'Linux office was not executed' )
Execute this code on a Windows auto:
Linux office was non executed
When an exception occurs in a plan running this function, the programme will continue as well as inform you lot most the fact that the function call was not successful.
What you did not get to see was the type of fault that was thrown every bit a result of the function call. In order to see exactly what went wrong, you would need to catch the error that the function threw.
The post-obit lawmaking is an example where yous capture the AssertionError
and output that message to screen:
try : linux_interaction () except AssertionError as mistake : print ( mistake ) print ( 'The linux_interaction() role was non executed' )
Running this function on a Windows car outputs the following:
Part can only run on Linux systems. The linux_interaction() function was non executed
The first bulletin is the AssertionError
, informing yous that the function tin merely be executed on a Linux machine. The 2nd message tells you which function was not executed.
In the previous example, you chosen a function that yous wrote yourself. When you executed the function, you caught the AssertionError
exception and printed information technology to screen.
Here'south another example where you open up a file and use a built-in exception:
try : with open ( 'file.log' ) as file : read_data = file . read () except : impress ( 'Could not open file.log' )
If file.log does not exist, this block of lawmaking will output the following:
This is an informative message, and our plan will withal go on to run. In the Python docs, you can see that there are a lot of built-in exceptions that you tin can utilise here. Ane exception described on that page is the following:
Exception
FileNotFoundError
Raised when a file or directory is requested but doesn't exist. Corresponds to errno ENOENT.
To take hold of this blazon of exception and impress it to screen, you could apply the post-obit code:
try : with open ( 'file.log' ) every bit file : read_data = file . read () except FileNotFoundError every bit fnf_error : print ( fnf_error )
In this case, if file.log does non exist, the output will be the following:
[Errno 2] No such file or directory: 'file.log'
Yous can have more one function call in your try
clause and anticipate catching diverse exceptions. A matter to notation here is that the code in the try
clause will stop as shortly as an exception is encountered.
Look at the following code. Here, y'all first phone call the linux_interaction()
function and and so effort to open a file:
attempt : linux_interaction () with open ( 'file.log' ) as file : read_data = file . read () except FileNotFoundError as fnf_error : impress ( fnf_error ) except AssertionError as fault : impress ( mistake ) print ( 'Linux linux_interaction() part was not executed' )
If the file does not be, running this code on a Windows automobile will output the following:
Role can only run on Linux systems. Linux linux_interaction() role was not executed
Inside the endeavor
clause, you lot ran into an exception immediately and did not become to the function where you effort to open file.log. Now await at what happens when y'all run the lawmaking on a Linux automobile:
[Errno two] No such file or directory: 'file.log'
Hither are the key takeaways:
- A
effort
clause is executed up until the signal where the starting time exception is encountered. - Inside the
except
clause, or the exception handler, yous determine how the program responds to the exception. - You lot tin can anticipate multiple exceptions and differentiate how the program should respond to them.
- Avoid using bare
except
clauses.
The else
Clause
In Python, using the else
statement, you can instruct a program to execute a sure block of code only in the absence of exceptions.
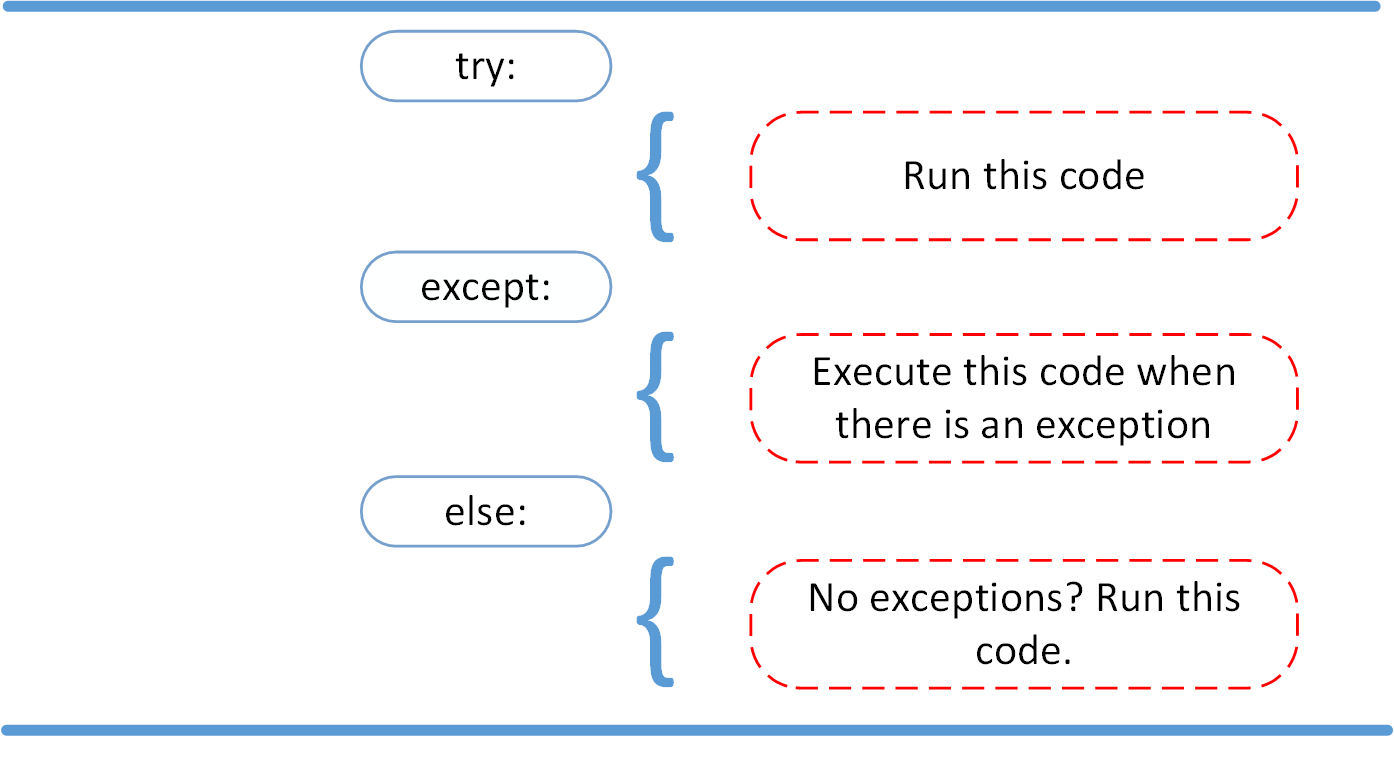
Wait at the post-obit case:
try : linux_interaction () except AssertionError as error : impress ( error ) else : print ( 'Executing the else clause.' )
If you lot were to run this code on a Linux system, the output would be the post-obit:
Doing something. Executing the else clause.
Because the program did not see any exceptions, the else
clause was executed.
Yous tin also try
to run code inside the else
clause and catch possible exceptions in that location as well:
try : linux_interaction () except AssertionError as error : print ( error ) else : try : with open ( 'file.log' ) as file : read_data = file . read () except FileNotFoundError as fnf_error : print ( fnf_error )
If y'all were to execute this code on a Linux machine, you would get the following result:
Doing something. [Errno ii] No such file or directory: 'file.log'
From the output, you lot can see that the linux_interaction()
function ran. Because no exceptions were encountered, an attempt to open file.log was made. That file did non exist, and instead of opening the file, you caught the FileNotFoundError
exception.
Cleaning Up Afterwards Using finally
Imagine that yous always had to implement some sort of activity to clean upwardly later on executing your code. Python enables you lot to exercise and so using the finally
clause.
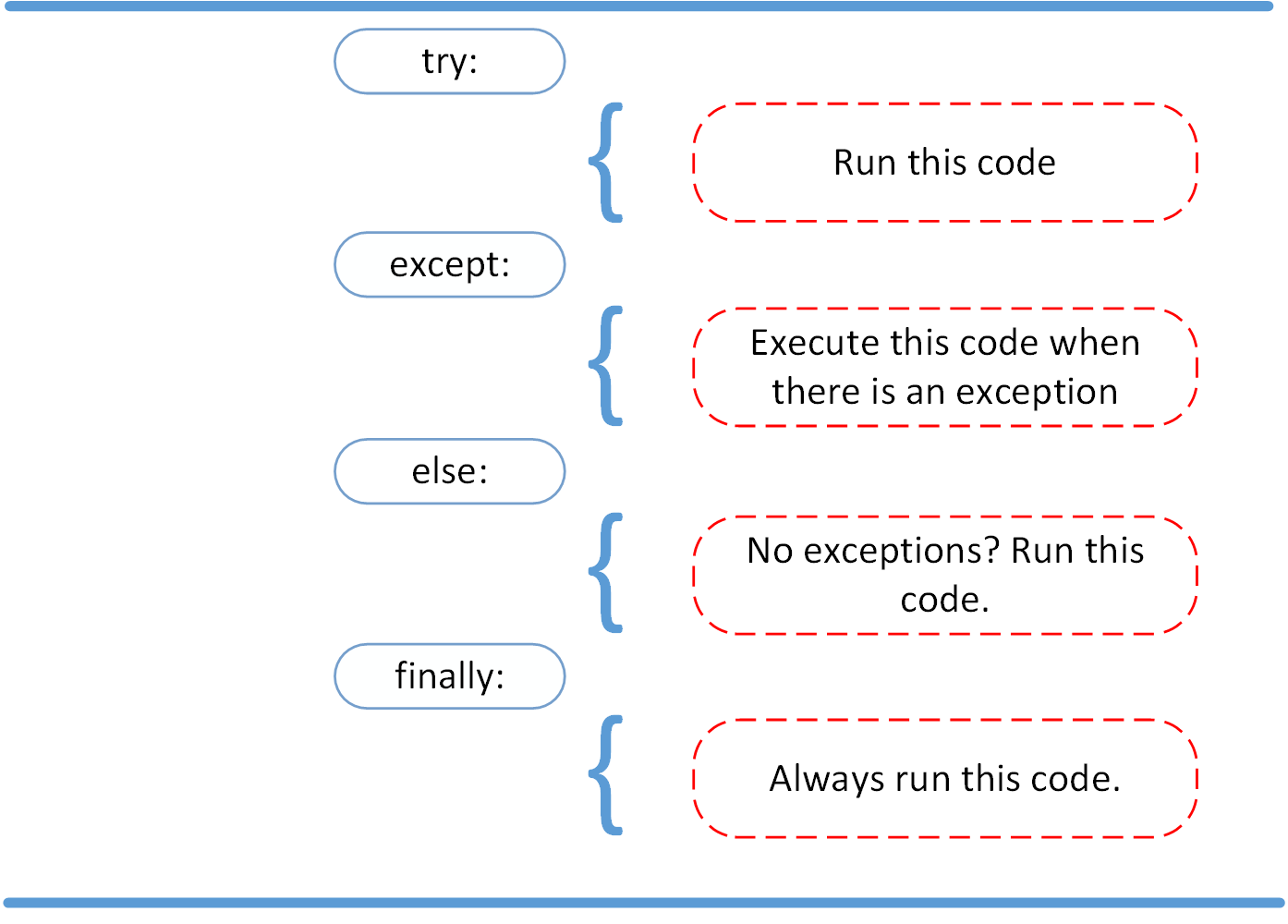
Have a look at the following example:
try : linux_interaction () except AssertionError as error : impress ( error ) else : attempt : with open ( 'file.log' ) as file : read_data = file . read () except FileNotFoundError as fnf_error : print ( fnf_error ) finally : impress ( 'Cleaning upward, irrespective of any exceptions.' )
In the previous code, everything in the finally
clause will exist executed. Information technology does not matter if yous encounter an exception somewhere in the attempt
or else
clauses. Running the previous code on a Windows car would output the following:
Role can only run on Linux systems. Cleaning upwards, irrespective of any exceptions.
Summing Up
After seeing the difference between syntax errors and exceptions, yous learned about various means to raise, catch, and handle exceptions in Python. In this article, you saw the following options:
-
raise
allows you to throw an exception at any time. -
assert
enables y'all to verify if a sure status is met and throw an exception if it isn't. - In the
try
clause, all statements are executed until an exception is encountered. -
except
is used to catch and handle the exception(due south) that are encountered in the try clause. -
else
lets you code sections that should run merely when no exceptions are encountered in the attempt clause. -
finally
enables yous to execute sections of code that should e'er run, with or without whatsoever previously encountered exceptions.
Hopefully, this commodity helped yous sympathise the basic tools that Python has to offering when dealing with exceptions.
Watch Now This tutorial has a related video class created by the Real Python team. Sentry it together with the written tutorial to deepen your understanding: Raising and Handling Python Exceptions
wickershaterinew2002.blogspot.com
Source: https://realpython.com/python-exceptions/
0 Response to "Python Raise Error and Ask Again"
Post a Comment